MongoDB | How to Optimize Queries
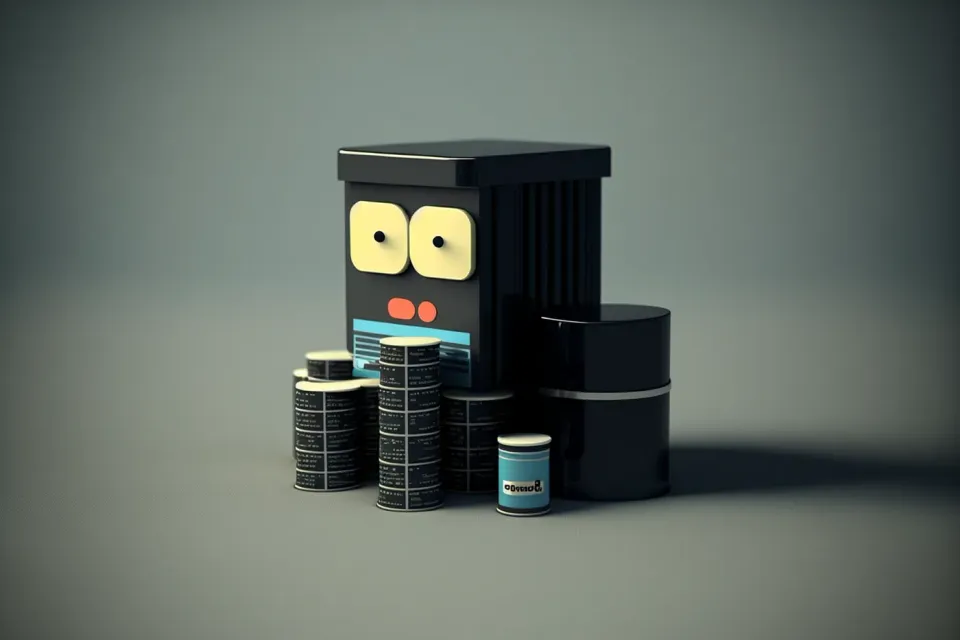
To optimize MongoDB queries, you can use the following techniques:
- Indexing: Create indexes on the fields you frequently search for to improve query performance.
- Query Optimization: Use the explain() method to analyze query performance and determine if additional indexes or other optimizations are needed.
- Projection: Limit the fields returned in a query to only the fields you need, reducing the amount of data transferred from the database to your application.
- Pagination: Use limit() and skip() to retrieve a subset of data and minimize the amount of data transferred.
- Caching: Use a caching layer, such as Redis, to store frequently used data and reduce the number of queries to the database.
- Proper Data Modeling: Store related data together in the same document to reduce the number of database queries needed to retrieve all the data needed for a single request.
- Use Proper Data Types: Use the proper data type for each field to reduce the size of data stored and improve query performance.
- Monitoring and Maintenance: Regularly monitor the performance of your database and take proactive measures to address potential performance issues before they become problems.
Indexing: Create indexes on the fields you frequently search for to improve query performance.
Indexing in MongoDB is a way to improve query performance by creating an index on one or more fields in a collection. When you create an index, MongoDB creates a data structure that stores the values of the indexed field(s) in a way that allows for fast and efficient searching.
For example, consider a collection of blog posts with the following structure:
{
_id: ObjectId(...),
title: "Hello World!",
body: "Lorem ipsum...",
tags: ["mongodb", "indexing"],
date: ISODate("2022-01-01T00:00:00.000Z")
}
If you frequently search for blog posts by their tags
, you can create an index on the tags
field to improve query performance:
db.posts.createIndex({ tags: 1 })
Now, when you search for blog posts with a specific tag, MongoDB can use the index to quickly find the relevant documents, rather than scanning the entire collection. For example:
db.posts.find({ tags: "mongodb" })
In this example, MongoDB can use the tags
index to quickly find all blog posts with the tag "mongodb". This makes the query much faster and more efficient than if MongoDB had to scan the entire collection to find the relevant documents.
Query Optimization: Use the explain() method to analyze query performance and determine if additional indexes or other optimizations are needed.
The explain()
method in MongoDB is used to analyze query performance and determine if additional indexes or other optimizations are needed. It provides information about how the query is executed, including the query plan, the number of documents scanned, and the number of documents returned.
For example, consider the following query:
db.posts.find({ tags: "mongodb" })
You can use the explain()
method to analyze the performance of this query:
db.posts.find({ tags: "mongodb" }).explain()
The output of the explain()
method will show the query plan that MongoDB used to execute the query, including information about how the query was optimized and which indexes were used (if any).
Here’s an example of the output of the explain()
method:
{
"queryPlanner" : {
"plannerVersion" : 1,
"namespace" : "blog.posts",
"indexFilterSet" : false,
"parsedQuery" : {
"tags" : {
"$eq" : "mongodb"
}
},
"winningPlan" : {
"stage" : "FETCH",
"inputStage" : {
"stage" : "IXSCAN",
"keyPattern" : {
"tags" : 1
},
"indexName" : "tags_1",
"isMultiKey" : false,
"multiKeyPaths" : {
"tags" : [ ]
},
"isUnique" : false,
"isSparse" : false,
"isPartial" : false,
"indexVersion" : 2,
"direction" : "forward",
"indexBounds" : {
"tags" : [
"[\"mongodb\", \"mongodb\"]"
]
}
}
},
"rejectedPlans" : [ ]
},
"executionStats" : {
"executionSuccess" : true,
"nReturned" : 2,
"executionTimeMillis" : 0,
"totalKeysExamined" : 2,
"totalDocsExamined" : 2,
"executionStages" : {
"stage" : "FETCH",
"nReturned" : 2,
"executionTimeMillisEstimate" : 0,
"works" : 3,
"advanced" : 2,
"needTime" : 0,
"needYield" : 0,
"saveState" : 0,
"restoreState" : 0,
"isEOF" : 1,
"invalidates" : 0,
"docsExamined" : 2,
"alreadyHasObj" : 0,
"inputStage" : {
"stage" : "IXSCAN",
"nReturned" : 2,
"executionTimeMillisEstimate" : 0,
"works" : 2,
"advanced" : 2,
"needTime" : 0,
"needYield" : 0,
"
Projection: Limit the fields returned in a query to only the fields you need, reducing the amount of data transferred from the database to your application.
Projection in MongoDB is a way to limit the fields returned in a query to only the fields that you need, reducing the amount of data transferred from the database to your application. This can improve query performance and reduce the amount of memory required to store the query results.
For example, consider a collection of blog posts with the following structure:
{
_id: ObjectId(...),
title: "Hello World!",
body: "Lorem ipsum...",
tags: ["mongodb", "indexing"],
date: ISODate("2022-01-01T00:00:00.000Z")
}
If you only need the title
and date
fields from the blog posts, you can use projection to limit the fields returned in the query:
db.posts.find({}, { title: 1, date: 1 })
In this example, the second argument to the find()
method specifies the projection, and the 1
values indicate that the title
and date
fields should be included in the results. The _id
field is included by default, so you don't need to include it in the projection.
This query returns the following results:
{
"_id" : ObjectId(...),
"title" : "Hello World!",
"date" : ISODate("2022-01-01T00:00:00.000Z")
}
Note that the body
and tags
fields are not included in the results, which reduces the amount of data transferred from the database to your application and improves query performance.
Pagination: Use limit() and skip() to retrieve a subset of data and minimize the amount of data transferred.
Pagination in MongoDB is a way to retrieve a subset of data by limiting the number of documents returned in a query and skipping a specified number of documents. This can be useful when you need to retrieve a large number of documents from a collection, but you only want to display a limited number of documents at a time.
For example, consider a collection of blog posts with the following structure:
{
_id: ObjectId(...),
title: "Hello World!",
body: "Lorem ipsum...",
tags: ["mongodb", "indexing"],
date: ISODate("2022-01-01T00:00:00.000Z")
}
To retrieve the second page of blog posts, where each page displays 10 posts, you can use the limit()
and skip()
methods:
db.posts.find({}).skip(10).limit(10)
In this example, the skip()
method skips the first 10 documents, and the limit()
method limits the number of documents returned to 10.
This query returns the following results:
[
{
"_id" : ObjectId(...),
"title" : "Hello World!",
"body" : "Lorem ipsum...",
"tags" : ["mongodb", "indexing"],
"date" : ISODate("2022-01-01T00:00:00.000Z")
},
...
]
Note that the limit()
method must be called after the skip()
method in order to ensure that the correct number of documents are returned. Using pagination in this way minimizes the amount of data transferred from the database to your application and improves query performance.
Caching: Use a caching layer, such as Redis, to store frequently used data and reduce the number of queries to the database.
Caching in MongoDB involves using a caching layer, such as Redis, to store frequently used data in memory, and reducing the number of queries to the database. This can improve the performance of your application by reducing the latency and load on the database.
For example, consider an e-commerce website that displays the top 10 products based on sales. The product data is stored in a MongoDB collection, and the sales data is stored in a separate collection.
To improve the performance of the website, you can use Redis to cache the top 10 products based on sales. Every time a sale is made, you update the Redis cache with the latest top 10 products.
Here’s an example of how you could implement this using Redis and Node.js:
const redis = require("redis");
const client = redis.createClient();
// Query MongoDB for the top 10 products based on sales
const getTopProducts = async () => {
const products = await db.products.aggregate([
{ $sort: { sales: -1 } },
{ $limit: 10 }
]);
return products;
};
// Store the top 10 products in the Redis cache
const setTopProductsCache = async () => {
const topProducts = await getTopProducts();
client.set("topProducts", JSON.stringify(topProducts));
};
// Retrieve the top 10 products from the Redis cache
const getTopProductsCache = () => {
return new Promise((resolve, reject) => {
client.get("topProducts", (err, data) => {
if (err) return reject(err);
resolve(JSON.parse(data));
});
});
};
// Get the top 10 products from the Redis cache if it exists, otherwise query MongoDB
const getTopProductsWithCache = async () => {
let topProducts;
try {
topProducts = await getTopProductsCache();
} catch (err) {
topProducts = await getTopProducts();
setTopProductsCache();
}
return topProducts;
};
In this example, the getTopProducts
function queries MongoDB for the top 10 products based on sales, the setTopProductsCache
function stores the top 10 products in the Redis cache, and the getTopProductsCache
function retrieves the top 10 products from the Redis cache. The getTopProductsWithCache
function gets the top 10 products from the Redis cache if it exists, and otherwise queries MongoDB.
By using a caching layer like Redis, you can reduce the number of queries to the database, which can improve the performance of your application.
Proper Data Modeling: Store related data together in the same document to reduce the number of database queries needed to retrieve all the data needed for a single request.
Proper data modeling in MongoDB involves designing the structure of your data to minimize the number of database queries needed to retrieve all the data needed for a single request. This can improve the performance of your application by reducing the latency and load on the database.
For example, consider a blogging platform that allows users to post articles and add comments. You could store the articles and comments in separate collections, but this would require two separate queries to retrieve all the data needed for a single article: one query to retrieve the article, and another query to retrieve the comments for that article.
A better approach would be to store the article and its comments in a single document, using a nested data structure. Here’s an example of what the document might look like:
{
"_id": ObjectId("5f8a7929ba24b82d0a9c38ed"),
"title": "How to Optimize MongoDB Queries",
"content": "Lorem ipsum dolor sit amet, consectetur adipiscing elit...",
"author": "John Doe",
"comments": [
{
"author": "Jane Doe",
"content": "Great article! I learned a lot.",
"date": ISODate("2023-01-01T12:00:00Z")
},
{
"author": "John Smith",
"content": "I agree. Very informative.",
"date": ISODate("2023-01-02T12:00:00Z")
}
],
"date": ISODate("2023-01-01T12:00:00Z")
}
In this example, the article and its comments are stored in a single document, using a nested array for the comments. By storing related data together in the same document, you can reduce the number of database queries needed to retrieve all the data needed for a single request, which can improve the performance of your application.
Use Proper Data Types: Use the proper data type for each field to reduce the size of data stored and improve query performance.
Using the proper data type for each field in MongoDB can help reduce the size of the data stored and improve query performance. By choosing the appropriate data type for each field, you can ensure that the data is stored in an efficient manner and can be queried quickly.
For example, consider a collection of user profiles that includes a field for the user’s date of birth. If the date of birth is stored as a string, it will require more space to store and will be slower to query, as MongoDB will need to perform a string comparison.
A better approach would be to store the date of birth as a Date
type. Here's an example of what the document might look like:
{
"_id": ObjectId("5f8a7929ba24b82d0a9c38ed"),
"name": "John Doe",
"email": "johndoe@example.com",
"dateOfBirth": ISODate("1980-01-01T12:00:00Z"),
"createdAt": ISODate("2023-01-01T12:00:00Z"),
"updatedAt": ISODate("2023-01-01T12:00:00Z")
}
In this example, the date of birth is stored as a Date
type, which is more compact and easier to query than a string representation. By using the proper data type for each field, you can reduce the size of the data stored and improve query performance.
Monitoring and Maintenance: Regularly monitor the performance of your database and take proactive measures to address potential performance issues before they become problems.
Monitoring and maintenance are important steps in ensuring the performance and reliability of your MongoDB database. Regularly monitoring the performance of your database can help you identify and resolve performance issues before they become critical.
For example, you might use MongoDB’s built-in db.serverStatus()
method to retrieve performance metrics, such as the number of read and write operations per second, the size of the data set, and the size of the working set (the portion of the data set that is frequently accessed). If you notice that the working set is larger than available memory, it may indicate that your system needs more memory, or that you need to optimize your data model.
In addition to monitoring performance, you should also regularly perform maintenance tasks, such as compacting data files, running repairs, and backing up your data. These tasks can help keep your database running smoothly and prevent data loss in the event of a failure.
Here’s an example of how you might perform a maintenance task in MongoDB:# Connect to the MongoDB server
# Connect to the MongoDB server
$ mongo
# Switch to the database you want to maintain
> use mydatabase
# Run the compact command
> db.runCommand({compact: "mycollection"})
In this example, we connect to the MongoDB server and switch to the database we want to maintain. We then run the compact
command, which compacts the data files for the mycollection
collection. By regularly performing maintenance tasks, you can ensure that your database is running efficiently and that your data is protected.
Member discussion