Nest.JS | Monads -> Maybe
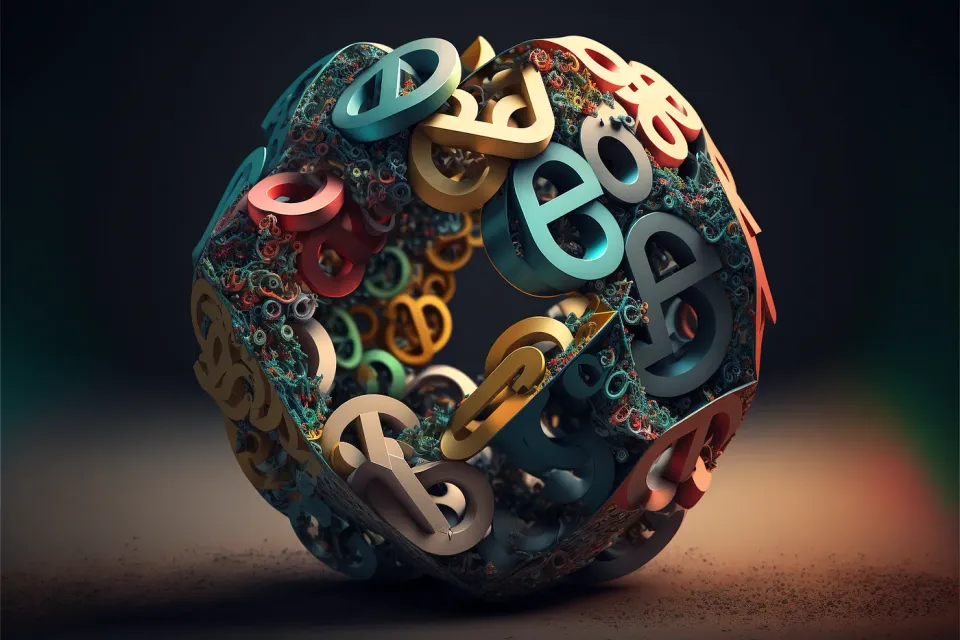
The Maybe monad, also known as the Option monad, is a monad that is used to represent computations that may or may not have a value. It is typically used to handle the possibility of null or undefined values in a functional and composable way.
The Maybe monad is typically implemented as an object that has two subtypes: Just
and Nothing
. The Just
subtype holds a value, while the Nothing
subtype represents the absence of a value.
Here’s an example of the Maybe monad in JavaScript:
class Just {
constructor(value) {
this.value = value;
}
map(f) {
return new Just(f(this.value));
}
chain(f) {
return f(this.value);
}
getOrElse(defaultValue) {
return this.value;
}
}
class Nothing {
map(f) {
return this;
}
chain(f) {
return this;
}
getOrElse(defaultValue) {
return defaultValue;
}
}
In this example, the Just
class takes a value in its constructor, and has methods map
, chain
and getOrElse
that allow you to transform the wrapped value and chain multiple computations together. The Nothing
class has the same methods but they don't do anything.
For example, you could create a Just
that wraps a number and then use the map
method to increment that number, you could then chain multiple of these Just
and Nothing
together to create a more complex computation, without having to manually check for the presence of a value at each step.
In Nest.js, the Maybe Monad is a Monad that wraps a value and provides a set of methods for working with that value in a functional way, while also handling the possibility of the value being null or undefined.
Here’s an example of how you could use the Maybe Monad in Nest.js:
import { Injectable } from '@nestjs/common';
import { Maybe, some, none } from 'fp-ts/lib/Maybe';
@Injectable()
export class MyService {
public findUserById(id: number): Maybe<User> {
const user = findUserInDB(id);
return user ? some(user) : none;
}
}
In this example, the MyService
class has a method findUserById(id: number)
that returns an instance of the Maybe
Monad, which wraps a User
object if one is found by the findUserInDB(id)
function, or none
if no user is found.
To extract the wrapped value, you can use the .getOrElse()
method and provide a default value to return if the wrapped value is none
console.log(myService.findUserById(1).getOrElse(new User()))
if the user with id 1 exists it will print the user’s details, otherwise it will print an empty user object
You can also use the .map()
function to transform the wrapped value if it exists, and .chain()
function to chain multiple maybe computations.
It’s important to note that this example is a basic one and doesn’t demonstrate the full power of Monads in functional programming. Monads can be used to handle various cases like error handling, chaining multiple computations and more, it’s best to understand the problem and use cases before applying monads.
Member discussion