Serverless | Easy start
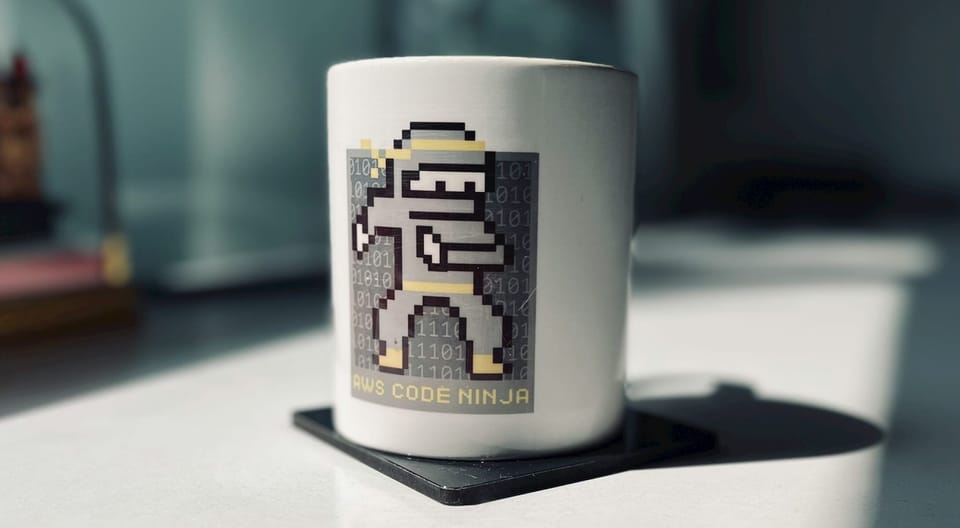
Serverless might sound like a world without servers, but that's not quite right. It's more about who manages the servers, not their complete absence. Here's the key idea:
Serverless is a cloud computing model where you focus on writing and deploying code, and the cloud provider handles the servers, scaling, and other infrastructure concerns.
Instead of provisioning and managing your own servers, you rely on the cloud provider's infrastructure and pay only for the resources your code uses. This simplifies development and allows you to scale your applications efficiently.
Here are some key features of serverless:
- Event-driven architecture: Your code executes in response to events, such as an API request, a database update, or a scheduled trigger. This means you don't need to continuously run servers waiting for requests.
- Automatic scaling: The cloud provider automatically scales your application up or down based on demand, so you don't have to worry about server capacity.
- Pay-per-use pricing: You only pay for the resources your code uses, making it cost-effective for applications with variable workloads.
Here are some common types of serverless services:
- Function as a Service (FaaS): Allows you to deploy code snippets that execute in response to events.
- Backend as a Service (BaaS): Provides pre-built backend functionality, such as user authentication and database management.
- API Gateway: Manages API requests and routes them to your serverless functions.
It's important to remember that serverless isn't a perfect fit for every situation. Here are some limitations to consider:
- Vendor lock-in: You may become locked into a specific cloud provider's serverless platform.
- Debugging challenges: Debugging serverless applications can be more complex than traditional server-based applications.
- Cold starts: The first time your code executes after a period of inactivity, it may take longer to start up.
Let's start build simple project via Serverless, DynamoDB
This app built with AWS Lambda, DynamoDB, and API Gateway. It is written in TypeScript and uses the Serverless Framework. The application includes features like user creation, shop creation, and email sending via AWS SES.
Prerequisites
- nodejs
- npm
- serverless framework
- aws cli
- aws credentials
- dynamodb local
- dynamodb admin
- dynamodb migrate
Features
- User
- Create a user
- Get a user
- Update a user
- Delete a user
- Shop
- Create a shop
- Get a shop
- Mail - send an email via AWS SES
Installation
Setup npm dependencies
npm install
Setup serverless framework
npm install -g serverless
Install dynamodb local
npx sls dynamodb install
Install dynamodb admin
npm install -g dynamodb-admin
after installation, run the following command to start dynamodb admin
dynamodb-admin
_note: admin will be available at http://localhost:8001
Install dynamodb migrate
npm install -g dynamodb-migrate
Run
npx serverless offline start --stage=dev
Deploy
npx serverless deploy --stage=<name>
Remove
npx serverless remove --stage=<name>
API Documentation
Will be available at http://localhost:3000/swagger
Project Structure
src
├── functions
│ ├── mail
│ │ ├── handler.ts
│ │ └── routes.ts
│ ├── shop
│ │ ├── handler.ts
│ │ └── routes.ts
│ └── user
│ ├── handler.ts
│ └── routes.ts
├── libs
│ ├── api-gateway.ts
│ ├── handler-resolver.ts
│ └── lambda.ts
├── model
│ ├── Shop.ts
│ ├── User.ts
│ └── index.ts
└── services
├── index.ts
├── shop-service.ts
└── user-service.ts
Each function has its own folder with a handler and routes file. The handler file contains the lambda function and the routes file contains the api gateway routes.
Example of the handler file:
const create = middyfy(
{
type: 'object',
required: ['body'],
properties: {
body: {
type: 'object',
required: ['email'],
properties: {
email: { type: 'string', format: 'email' },
},
},
},
},
async (event: APIGatewayProxyEventWithBody<any>): Promise<APIGatewayProxyResult> => {
const user = await userService.create({
email: event.body.email,
userId: uuidv4(),
isVerified: false,
});
return formatJSONResponse({
user,
});
},
);
middify
- is a helper function that wraps the lambda function with params validation and error handling.
If you have any questions or feedback about this article, feel free to leave a comment.
Thanks for reading and let's start working together.
Member discussion